How to create Menu Button Expand To Navigation Menu On Click using html css and js. Designed by Mathieu Lavoie a codepen user.
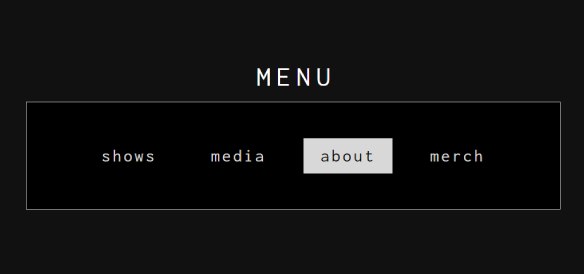
HTML
<!DOCTYPE html> <html lang="en" > <head> <meta charset="UTF-8"> <title>CodePen - A Pen by Mathieu Lavoie</title> <link rel="stylesheet" href="./style.css"> </head> <body> <!-- partial:index.partial.html --> <div class="menuContainer"> <span class="menuTitle">menu</span> <div class="menu"> <ul> <li> <a href="#">shows</a></li> <li> <a href="#">media</a></li> <li> <a href="#">about</a></li> <li> <a href="#">merch</a></li> </ul> </div> </div> <!-- partial --> <script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.4/jquery.min.js"></script> <script src='https://cdnjs.cloudflare.com/ajax/libs/gsap/2.1.3/TweenMax.min.js'></script> <script src="./script.js"></script> </body> </html>
CSS
@import url(https://fonts.googleapis.com/css?family=Inconsolata:400,700); body { background: #111; color: #D8D8D8; } * li { list-style: none; } * a { text-decoration: none; color: #D8D8D8; } .menuContainer { display: block; position: relative; width: 750px; margin: 0 auto; } .menu { width: 150px; height: 150px; display: block; position: relative; margin: 0px auto; background: #D8D8D8; border-radius: 100%; border: 1px solid #D8D8D8; cursor: pointer; z-index: 10; } .menu ul { padding: 0; width: 100%; display: none; position: relative; text-align: center; top: 35px; } .menu ul li { display: inline-block; position: relative; font-family: Inconsolata; font-size: 25px; margin-right: 25px; box-sizing: border-box; width: 125px; letter-spacing: 3px; text-align: center; line-height: 50px; } .menu ul li:active { text-transform: uppercase; } .menu ul li:last-child { margin-right: 0; } .menu ul li:hover { background: #D8D8D8; } .menu ul li:hover a { color: #000; } .menuTitle { line-height: 150px; width: 150px; color: #000; text-transform: uppercase; font-size: 40px; text-align: center; letter-spacing: 8px; display: block; position: relative; margin: 0 auto; top: 150px; z-index: 11; padding-left: 10px; font-family: Inconsolata; cursor: pointer; -webkit-user-select: none; }
JAVASCRIPT
var $menu = $('.menu'); var $wholeMenu = $('.menu, .menuTitle'); var $menuUl = $('.menu ul'); var $menuTitle = $('.menuTitle'); var $menuContainer= $('.menuContainer'); var count = 0; var click = true; $wholeMenu.click(function(){ if (click) { click = false; $menuTitle.css({'z-index': 9}); var tl = new TimelineLite(); tl.to($menu, 0.4, {css:{ width: 750, background: "#000", borderRadius: 0, borderTop: "1px solid #D8D8D8" }, ease:Expo.easeInOut}); tl.to($menuTitle, 0.25, {x:0, y:-110, color:"#fff"}); setTimeout(function(){ $menuUl.fadeIn(); }, 250); } else { click = true; $menuTitle.css({'z-index': 11}); $(this).css({borderRadius: "100%"}) var tl = new TimelineLite(); tl.to($menu, 0.25, {css:{ width: 150, background: "#FFF", borderRadius: "100%", borderTop: "1px solid #D8D8D8" }, ease:Expo.easeInOut}) tl.to($menuTitle, 0.25, {x:0, y:0, color:"#000"}); $menuUl.hide(); }; })